This course will help you master essential data structures in Python, enabling you to write efficient code, optimize memory, and solve complex problems. By the end, you’ll be proficient in implementing arrays, linked lists, stacks, queues, trees, and graphs, along with recursion, searching, and sorting techniques.

Cultivate your career with expert-led programs, job-ready certificates, and 10,000 ways to grow. All for $25/month, billed annually. Save now
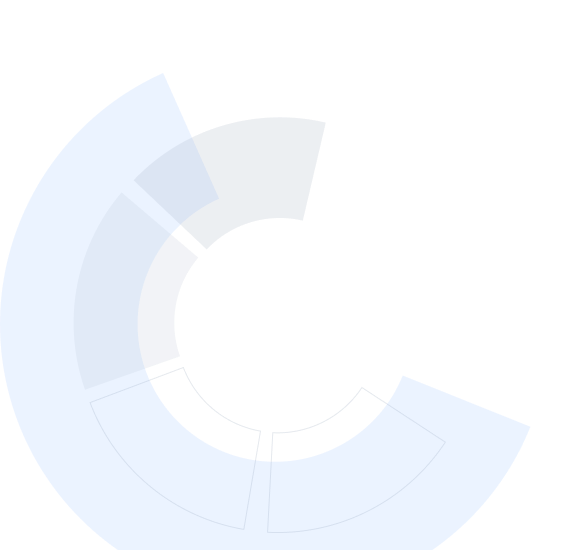
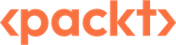
Recommended experience
Recommended experience
What you'll learn
Efficiently implement and manipulate arrays, linked lists, stacks, and queues in Python.
Develop recursive algorithms and work with binary trees and their traversals.
Analyze and solve graph-based problems using adjacency matrices and lists.
Details to know

Add to your LinkedIn profile
February 2025
9 assignments
See how employees at top companies are mastering in-demand skills
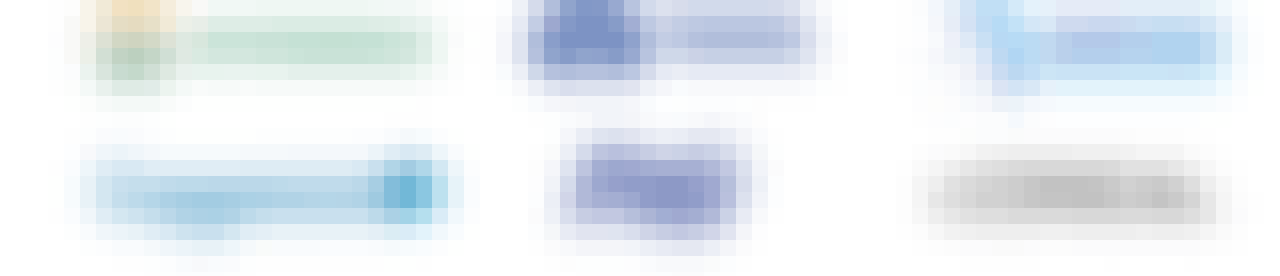
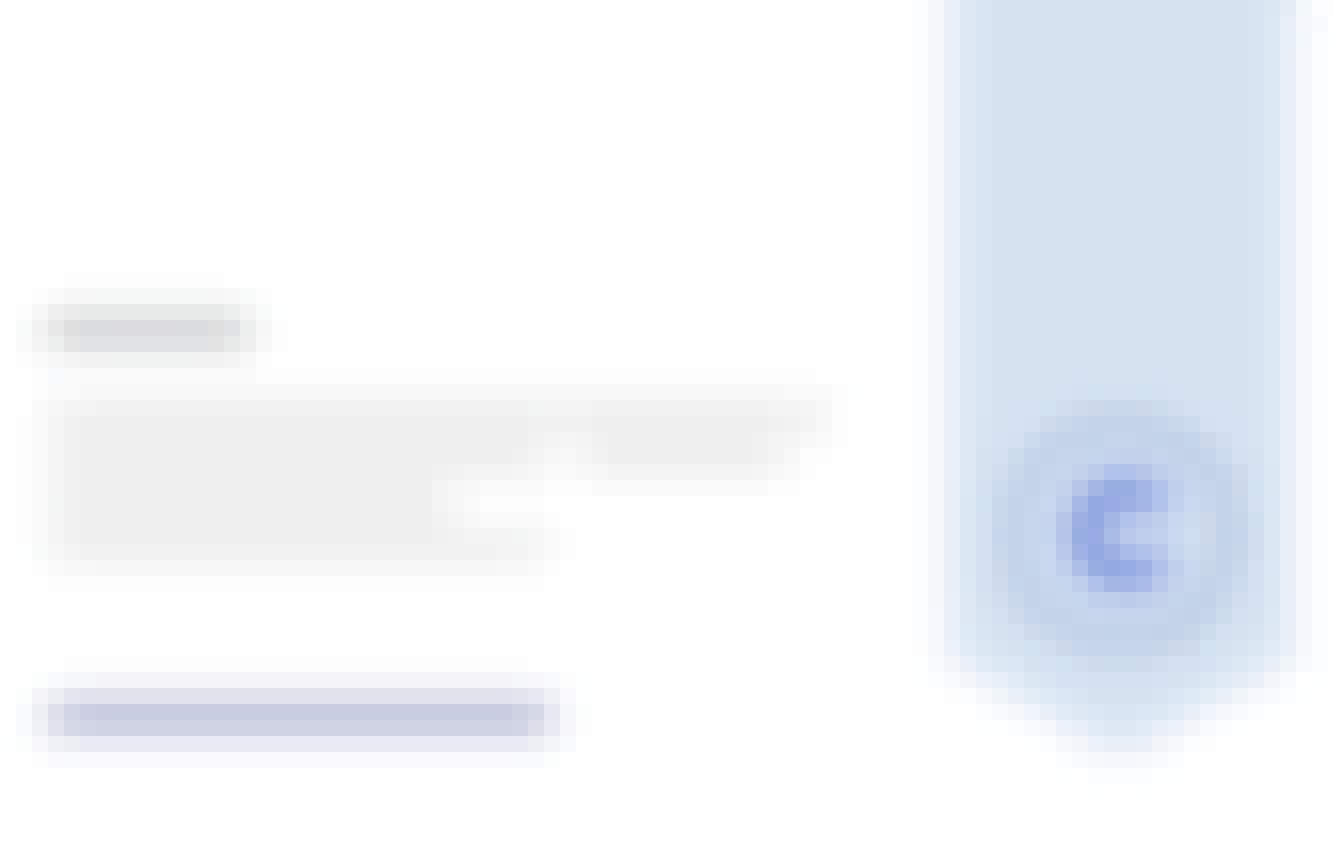
Earn a career certificate
Add this credential to your LinkedIn profile, resume, or CV
Share it on social media and in your performance review
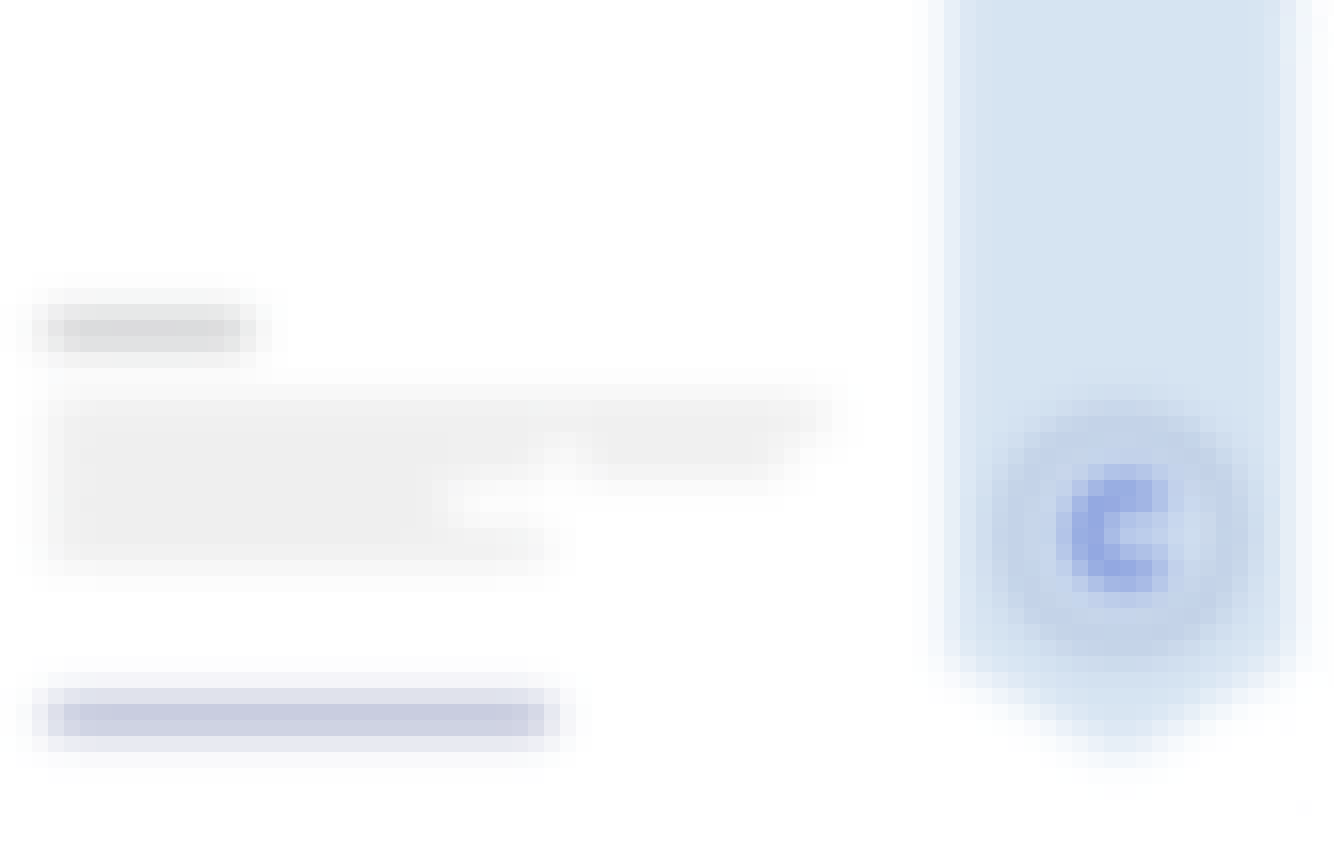
There are 9 modules in this course
In this module, we will explore the fundamentals of arrays, including their creation, manipulation, and applications. Starting with 1D arrays, we will cover essential operations such as searching, insertion, deletion, and updating. Then, we will progress to 2D arrays, learning how to access, insert, delete, and update elements, equipping you with the skills to handle data in Python effectively.
What's included
11 videos1 reading
In this module, we will dive into Python's versatile data structures: lists, tuples, sets, and dictionaries. You will learn to perform operations like accessing, modifying, and extending lists, as well as unpacking tuples and managing dictionary key-value pairs. This section also introduces set operations, preparing you for efficient data storage and retrieval techniques.
What's included
24 videos1 assignment
In this module, we will cover the essentials of recursion, starting with function basics and progressively building towards recursive solutions. You will learn how to design, implement, and debug recursive algorithms, gaining insights into their utility in solving problems like factorial computation and tree traversal.
What's included
5 videos1 assignment
In this module, we will delve into linked lists, a dynamic data structure suited for efficient memory usage. You will learn to perform operations such as insertion, deletion, and searching, along with advanced techniques like identifying the middle element and comparing linked lists. This module concludes with memory management techniques like deleting a linked list.
What's included
7 videos1 assignment
In this module, we will explore stacks, a fundamental data structure. You will learn various implementation methods using Python's list, collections, and queue modules. Additionally, you will apply stack operations to solve problems such as ensuring balanced parentheses, preparing you for practical programming challenges.
What's included
8 videos1 assignment
In this module, we will study queues and their variants, including circular queues. You will learn how to implement queue operations using Python's built-in methods and modules. The focus will also include optimizing space and time complexities for sequential data management.
What's included
6 videos1 assignment
In this module, we will explore tree structures, starting with basic terminology and progressing to binary trees. You will learn traversal techniques and implement operations to compute height and sum elements. This module lays the groundwork for understanding hierarchical data organization.
What's included
9 videos1 assignment
In this module, we will focus on binary search trees, a specialized tree structure optimized for searching and data organization. You will learn how to create, search, and insert nodes into a BST, preparing you for applications that require fast and ordered data handling.
What's included
4 videos1 assignment
In this module, we will introduce graphs, one of the most versatile data structures in computer science. You will learn to represent graphs using adjacency matrices and lists, equipping you with the tools to understand and implement graph-based algorithms for real-world problems.
What's included
3 videos2 assignments
Instructor

Offered by
Recommended if you're interested in Software Development
Coursera Project Network
University of Michigan
University of Colorado Boulder
Why people choose Coursera for their career




New to Software Development? Start here.

Open new doors with Coursera Plus
Unlimited access to 10,000+ world-class courses, hands-on projects, and job-ready certificate programs - all included in your subscription
Advance your career with an online degree
Earn a degree from world-class universities - 100% online
Join over 3,400 global companies that choose Coursera for Business
Upskill your employees to excel in the digital economy
Frequently asked questions
Yes, you can preview the first video and view the syllabus before you enroll. You must purchase the course to access content not included in the preview.
If you decide to enroll in the course before the session start date, you will have access to all of the lecture videos and readings for the course. You’ll be able to submit assignments once the session starts.
Once you enroll and your session begins, you will have access to all videos and other resources, including reading items and the course discussion forum. You’ll be able to view and submit practice assessments, and complete required graded assignments to earn a grade and a Course Certificate.
More questions
Financial aid available,